By Alx: October 2020 | last update: October 2020
Spring Boot REST API Documentation 'code first'
with SpringFox
Goal
Let's assume you have already a spring boot project(Code first approach) which almost finished and now the customer or test guys want to use the sawgger file. With SpringFox you have a tool that helps creating swagger documentation from existing code.What is SpringFox
The Springfox suite of java libraries are all about automating the generation of machine and human readable specifications for JSON APIs written using the spring family of projects
What we need
Java 11Maven 3.x
SpringFox dependency
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
After adding the dependency we'll restart the application and check the swagger-ui page, which be available at http://localhost:8080/swagger-ui/index.html
At first sight it seems to be okay, springfox renders the new page and there we can see the API. But there are some weird stuffs, such as the basic-error-controller
or the servers drop-down list with "Inferred Url".
With just a small change in pom file (added one dependency) you got the swagger-ui page with basic presentation, is a fine thing. Now we need to add some configuration.
Configuration
@Configuration
@EnableOpenApi
public class DocumentationrConfig {
@Bean
public Docket api() {
Server serverLocal = new Server("local", "http://localhost:8080", "for local usages", Collections.emptyList(), Collections.emptyList());
Server testServer = new Server("test", "https://example.org", "for testing", Collections.emptyList(), Collections.emptyList());
return new Docket(DocumentationType.OAS_30)
.servers(serverLocal, testServer)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.tutorial.codefirst"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("Openapi OAS3 with springfox ")
.description("Code first approach")
.version("1.0.0")
.contact(new Contact("Marone", "https://wstutorial.com", "test@wstutorial.com"))
.build();
}
}
The class is marked with @Configuration
@EnableOpenApi
enables the usage of OpenAPI(OAS3)
Docket is the primary interface into the Springfox framework, here is initialized for OpenAPI 3
The ApiInfo delivers api's meta information such as: title, contact, version, etc
The servers should set up the servers (local and test environment), but it seems to be buggy at the moment (Version 3.0.0), we will show a workaround later.
Documenting the RestController
Config Get all
Before: After: We add two Annotations @ApiOperation
and @ApiResponse
, but there are not really necessary. Springfox generates by default all the needed documentation for all HTTP verbs.
In case of GET, there are four default responses:
- 200 -> OK
- 401 -> Unauthorized
- 403 -> Forbidden
- 404 -> Not Found
Documenting the model
Workaround
This solution was inspired by rowleyn, now the whole swagger-ui page looks fine
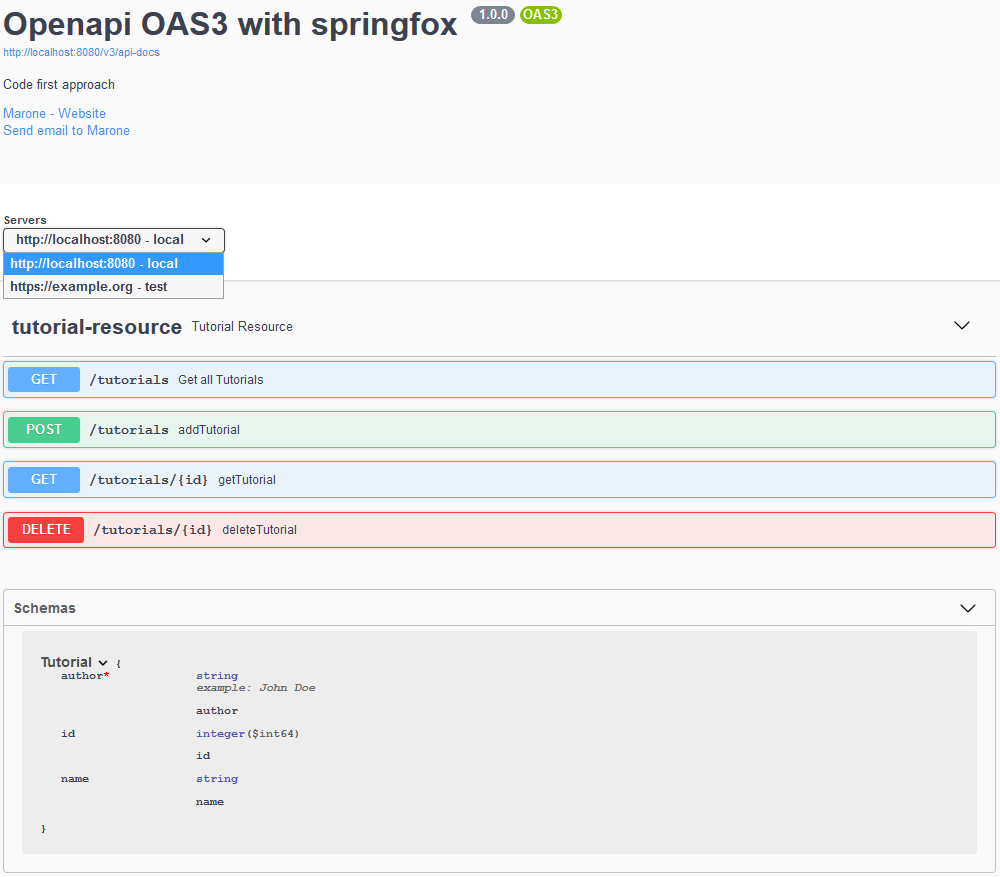