By Marone: July 2016
Hello world REST API with jersey
Goal
This article describes how to create a simple rest web service without using a web container (stand alone).It uses the Java BuiltIn HttpServer and JAX-RS.Used technologies
JDK 1.8Maven 3.2
Maven dependencies: jersey 1.18.4
pom.xml
<dependencies>
<dependency>
<groupId>com.sun.jersey</groupId>
<artifactId>jersey-bundle</artifactId>
<version>1.18.4</version>
</dependency>
</dependencies>
Resource: HelloWorld
package com.wstutorial.rest;
import javax.ws.rs.*;
import javax.ws.rs.core.MediaType;
@Path("hello")
public class HelloWorldResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String message() {
return "hello World! ";
}
}
Rest Starter
package com.wstutorial.rest;
import com.sun.jersey.api.container.httpserver.HttpServerFactory;
import com.sun.net.httpserver.HttpServer;
public class StartRestServer {
public static void main(String[] args) {
HttpServer server;
try {
server = HttpServerFactory.create( "http://localhost:10080/api" );
server.start();
}catch (Exception e) {
System.out.println("Errormessage : " + e.getMessage());
}
}
}
Call the url
http://localhost:10080/api/helloShow the WADL
Hit the url: http://localhost:10080/api/application.wadl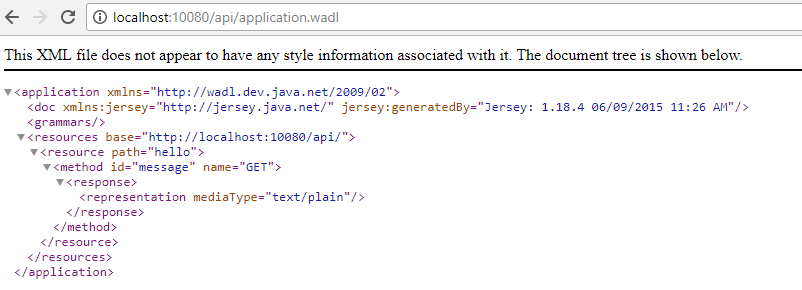
Important
Since Jersey 2.5.1 the WADL generated by default is WADL in shorter form without additional extension resources (OPTIONS methods, WADL resource). In order to get full WADL use the query parameter detail=true
Since Jersey 2.5.1 the WADL generated by default is WADL in shorter form without additional extension resources (OPTIONS methods, WADL resource). In order to get full WADL use the query parameter detail=true