By Marone: September 2020 | last update: October 2020
Generate code for Spring boot project
using openapi generator
Table of contents
- Goal
- What is OpenAPI Generator?
- What we need
- Use OpenAPI Generator CLI
- Using Maven Plugin
- Conclusion
- References
Goal
After designing REST API with openapi specification, now we will learn how to generate code from the defined openapi file. To achieve that, We will use the OpenAPI Generator and exactly we'll use the Server generator for spring. The output will be a spring boot maven project.What is OpenAPI Generator?
OpenAPI Generator is a open-source project to generate REST API clients, server stubs and documentation from on an OpenAPI specification (fka Swagger specification) document.
There are a number of ways to use OpenAPI Generator:
- Openapi generator cli
- With Plugins (Maven or Gradle)
- Online(either publicly hosted or self-hosted)
What we need
Java 11Maven 3.x
openapi-generator-cli 4.3.1
Use OpenAPI Generator CLI
OpenAPI Generator's CLI is a command-line tool.
Get the CLI
In Order to generate classes we need the cli jar FilePerpare the config file
{
"basePackage": "com.tutorial.codegen",
"configPackage": "com.tutorial.codegen.config",
"apiPackage": "com.tutorial.codegen.controllers",
"modelPackage": "com.tutorial.codegen.model",
"groupId": "com.tutorial",
"artifactId": "spring-boot-codegenerator"
}
In the conf.json
file are names of packages (for model, controllers and config) defined, groupId
and artifactId
will take place in the generated pom.xml
Run the command
java -jar openapi-generator-cli-4.3.1.jar generate -g spring -i openapi.yaml -c conf.json -o spring-boot-codegenerator
We run the jar with command generate, -g spring
indicates that we use spring as generator. We pass the openapi specification openapi.yaml
and the config conf.json
file too.
The last argument is for the output directory-o spring-boot-codegenerator
Project structure
The generated spring boot project looks like:spring-boot-codegenerator/
|-- src/
| |-- main/
| | |-- java/
| | | |-- com.wstutorial.codegen/
| | | | |-- config/
| | | | | |-- HomeController.java
| | | | | |-- OpenAPIDocumentationConfig.java
| | | | |-- controllers/
| | | | | |-- ApiUtil.java
| | | | | |-- TutorialsApi.java
| | | | | |-- TutorialsApiController.java
| | | | |-- model/
| | | | | |-- Tutorial.java
| | | | |-- OpenAPI2SpringBoot.java
| | | | |-- RFC3339DateFormat.java
| | |-- resources/
| | | |-- application.properties
|-- .openapi-generator-ignore
|-- pom.xml
|-- README.md
Start the project
mvn package spring-boot:run
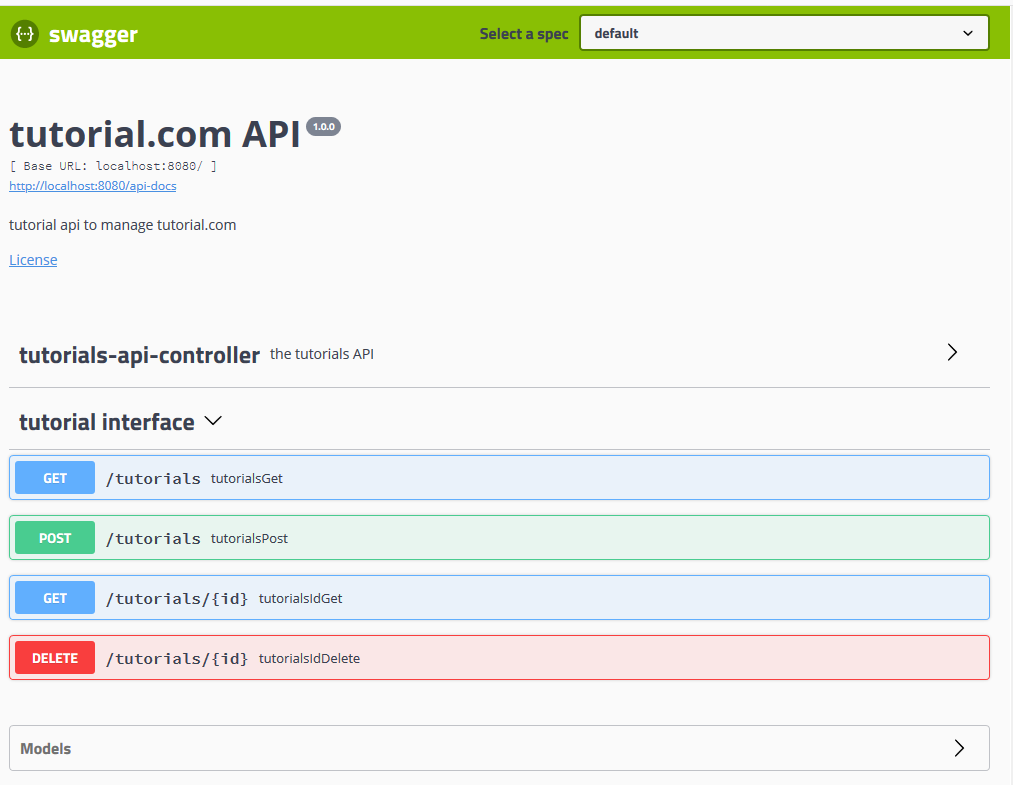
Call the API
$ curl -i http://localhost:8080/tutorials/2
HTTP/1.1 501
Content-Type: application/json;charset=UTF-8
Content-Length: 50
Date: Mon, 28 Sep 2020 04:35:05 GMT
Connection: close
{ "author" : "author", "name" : "name", "id" : 0 }
We get a response with dummy data but the http status code is 500, which means Not Implemented
. Now we can implement the business logic.
Using Maven Plugin
At first we need a empty spring boot maven project.Create an empty project
Go to Spring Initializr and create a demo maven project as following: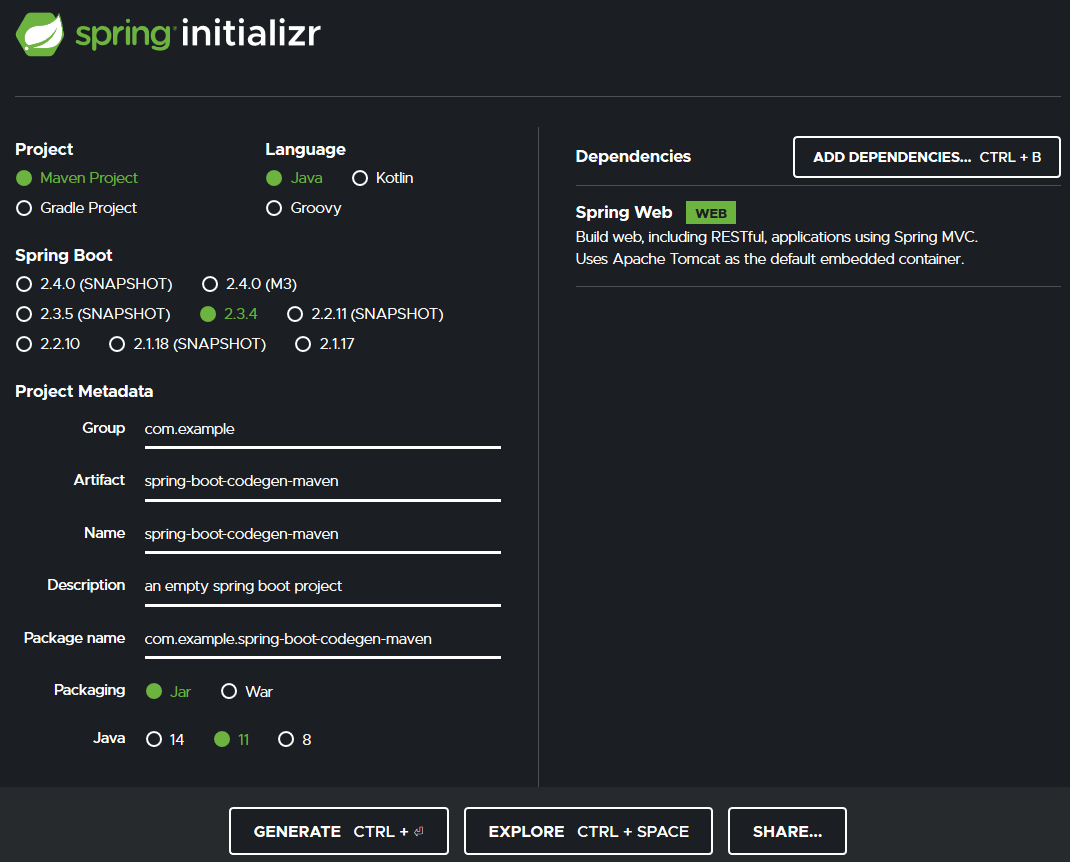
The openapi.yaml file was copied under
src/main/resources/openapi/openapi.yaml
.
Prepare the pom file
Unlike the cli case, we want to prevent the Docket configuration class from being generated. For that reason we added the option interfaceOnly = true
interfaceOnly = true
the generator was trying to generate a OpenAPIDocumentationConfig class.
We are not sure if this way the best one to solve this "challenge", but it worked for us. If you have a better solution, please let us know.
Generate code and check the output
Just run the command mvn package.The generator will generate the endpoint TutorialsApi
class, the model class Tutorial
and a Helper class ApiUtil
. The generated code are located under target
folder.
spring-boot-codegenerator/
|-- src/
| |-- main/
| | |-- resources/
| | | |-- openapi/
| | | | |-- openapi.yaml
|-- target/
| |-- generated-sources/
| | |-- openapi/
| | | |-- src/
| | | | |-- main/
| | | | | |-- java/
| | | | | | |-- com.wstutorial.codegen/
| | | | | | | |-- model/
| | | | | | | | |-- Tutorial.java
| | | | | | | |-- ApiUtil.java
| | | | | | | |-- TutorialsApi.java
Conclusion
As we have seen, it took really a few miniutes to create a whole spring boot project or to generate all the boilerplate code from a single openapi specification file. The development team can start implementing the business logic.
There is only one thing we want to point out, during using openapi-generator-cli the swagger docket configuration was pointing the version 2 (aka SWAGGER_2) and not the version specified in the specification file. May be something can be easily fixed with some configuration arguments?
Let's Summarize, using the openapi generator is easy. You can set up a project in a short time. It is a great tool and offers a lot of features. We love it.