By Alx: December 2017 | last update: December 2019
JAXWS top-down approach with tomcat
Goal
In a previous topic we learned how to develop JAX-WS web service With Top-Down approach (contract first). The service was published withjavax.xml.ws.Endpoint
. But in Real World you want to deploy your services in a servlet engine or maybe in application server :-). So now we are going to explain how to deploy our last example in Apache Tomcat
Used technologies
JDK 1.8Apache Tomcat 8.0.47
Maven 3.2
Eclipse IDE
Create maven project in eclipse
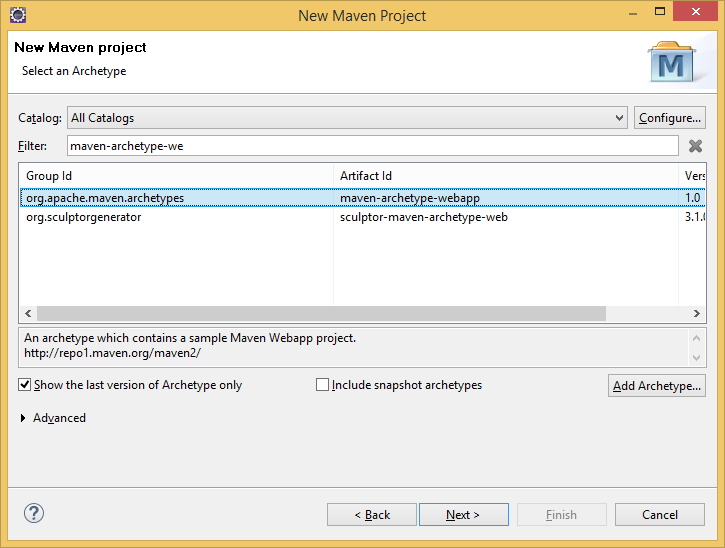
After finising the wizrad, make sure that
targeted runtime
is pointing Tomcat
Generate code
At first copy wsdl folder that contains wsdls and xsd files from previous article and execute the following command
wsimport -d ./generated -s ./src/main/java -p com.wstutorial.ws ./wsdl/TutorialService.wsdl
Now copy the TutorialServiceImpl.java
and your project should be error-free
Adjust the pom file
<dependency>
<groupId>com.sun.xml.ws</groupId>
<artifactId>jaxws-rt</artifactId>
<version>2.2.10</version>
</dependency>
Info! The depenedencies of
If you want to use embedded tomcat server in eclipse, you have to copy JAXWS RI jars to WEB-INF\lib
jaxws-rt
are not visibile in eclipse project structure, the will be only affected during maven build cycle mvn package
If you want to use embedded tomcat server in eclipse, you have to copy JAXWS RI jars to WEB-INF\lib
Perpare deployment
I) web.xml
The web.xml should look like below.
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<listener>
<listener-class>com.sun.xml.ws.transport.http.servlet.WSServletContextListener</listener-class>
</listener>
<servlet>
<servlet-name>TutorialService</servlet-name>
<servlet-class>com.sun.xml.ws.transport.http.servlet.WSServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>TutorialService</servlet-name>
<url-pattern>/tutorialService</url-pattern>
</servlet-mapping>
</web-app>
II) sun-jaxws.xml
Create a file named sun-jaxws.xml in WEB-INF folder and change it like below.
<?xml version="1.0" encoding="UTF-8"?>
<endpoints xmlns="http://java.sun.com/xml/ns/jax-ws/ri/runtime" version="2.0">
<endpoint
name="TutorialServiceImpl"
implementation="com.wstutorial.ws.impl.TutorialServiceImpl"
url-pattern="/tutorialService"/>
</endpoints>
III) build and deploy
mvn clean package
Copy target/ws-topdown-tomcat.war to %TOMCAT_HOME%/webapps and start tomcat
Check the url
http://localhost:18080/ws-topdown-tomcat/tutorialServicehttp://localhost:18080/ws-topdown-tomcat/tutorialService?wsdl