By Marone: August 2020
Secure API behind
Spring Cloud Gateway with apikey
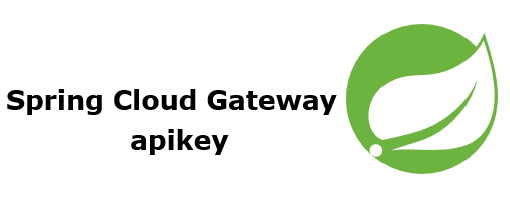
Table of contents
- Goal
- What is API Key?
- How to pass an apikey?
- Used tools
- The custom Filter
- Use the Filter
- let's test
- References
Goal
Sometimes there a need to secure an API with API key. When I started looking for examples about how spring cloud gateway deal with apikey, I found some articles but no examples. So we decide to write this short article. If it were up to me, I would always prefer OAuth2. But sometimes there are cases where the customer wants something easy and simple for authentication. Furthermore, there are many public APIs that use an apikey, such as Google Maps, Twitter,..etc. And I can tell you the list was long.What is API Key?
An application programming interface key (API Key) is a unique piece of code used to authenticate the calling user, developer, or application.Basically used to limit and monitor the usages of an API. The API keys are part of the request and therefore less secure. If you want to take a look at how the combination between API Gateway and OAuth2 works, here is an example of how KONG deals with it.
How to pass an apikey?
An API key can then be passed through:- A query string parameter,
- HTTP request headers,
- or cookie
Used tools
Java 11Maven 3.x
cURL 7.x
The custom Filter
Spring Cloud Gateway provides out of the box a lot of filters, but in this case we will write a custom filter. Custom filters have to implement the GatewayFilter and Ordered interfaces.
The
getOrder()
method is used to set the order of the filter. The filter method is part of GatewayFilter and mostly used to implement some cross-cutting logic. In our case it will check if the caller is allowed to consume the downstream service. This logic should be executed before the request passed. Generally speaking, we implemented a Prefilter.
In the example the filter is used to extract the apikey from header and check if is valid. If the apikey is not present or not match the dummy data the filter returns 401 status code.
Please keep in mind this example is made simple just to demonstrate how to secure an API with apikey and how powerful filters are.
The
checkApikey(String routeId, String apikey)
method is straightforward, here you can impelement some logic to get data from store and compare it with the given apikey. The custom filter can also read the name of the route routeId
Use the Filter
Now we need to register the ApiKeyFilter to the routelet's test
Curl command
When passing a appropriate apikey the request will be forwarded tohttpbin.org
The Gatewayfilter extracts the header and checks if the consumer is allowed to call the downstream service. In this case, the authentication was successful and we got a response from
httpbin.org
Curl command without apikey
Now testing without header, the api gateway respond with401 Unauthorized
References
The complete code can be found in GitHub