By Alx: October 2020 | last update: October 2020
Spring Boot REST API Documentation
with SpringDoc
Goal
In the previous article, we have seen how to document an existing REST API with SpringFox. It was pretty straight forward. Now let us discover another library springdoc-openapi, a java library for automating the generation of API documentation using spring boot project
What is SpringDoc
springdoc-openapi java library helps automating the generation of API documentation using spring boot projects. springdoc-openapi works by examining an application at runtime to infer API semantics based on spring configurations, class structure and various annotations
What we need
Java 11Maven 3.x
SpringDoc dependency
Now let's add the following dependency into Maven <dependency>
<groupId>org.springdo</groupId>
<artifactId>springdoc-openapi-ui</artifactId>
<version>1.4.8</version>
</dependency>
Now we run the Spring Boot application and visit the following URL: http://localhost:8080/swagger-ui.html, the browser will redirect it to http://localhost:8080/swagger-ui/index.html?configUrl=/v3/api-docs/swagger-config
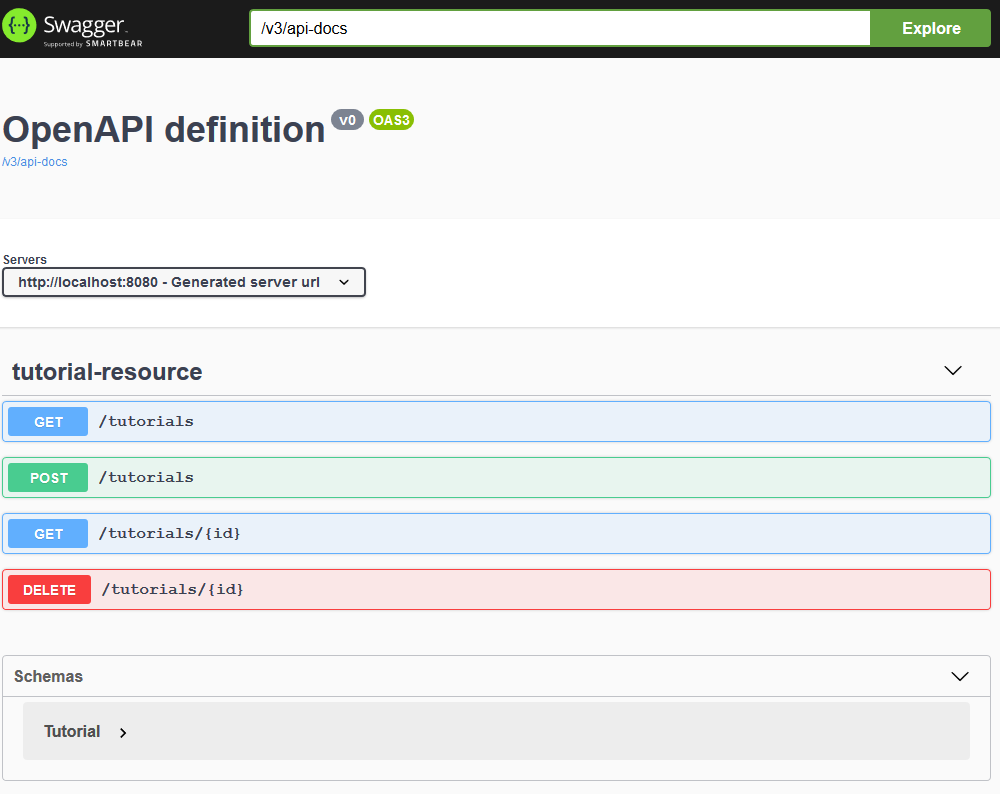
The swagger-ui page reflects our code, no more and no less. If you want more details you have to add more annotations.
Configuration
@Configuration
public class DocumentationConfig {
@Bean
public OpenAPI openAPI() {
Server localServer = new Server();
localServer.setDescription("local");
localServer.setUrl("http://localhost:8080");
Server testServer = new Server();
testServer.setDescription("test");
testServer.setUrl("https://example.org");
OpenAPI openAPI = new OpenAPI();
openAPI.info(new Info()
.title("Tutorial Rest API")
.description(
"Documenting Spring Boot REST API with SpringDoc and OpenAPI 3 spec")
.version("1.0.0")
.contact(new Contact().name("Marone").
url("https://wstutorial.com").email("test@wstutorial.com")));;
openAPI.setServers(Arrays.asList(localServer, testServer));
return openAPI;
}
}
The class is marked with @Configuration
, now spring will read the @Bean on runtime
OpenAPI
is the main document to describe the API, it provides meta informations such as: title, contact,etc.
The OpenAPI class is part of The OAS (OpenAPI Specification) standard
Documenting the RestController
Now, let's change the first operation in the RestController.Before: After:
Documenting the model
After restarting the application, we can see more informations:
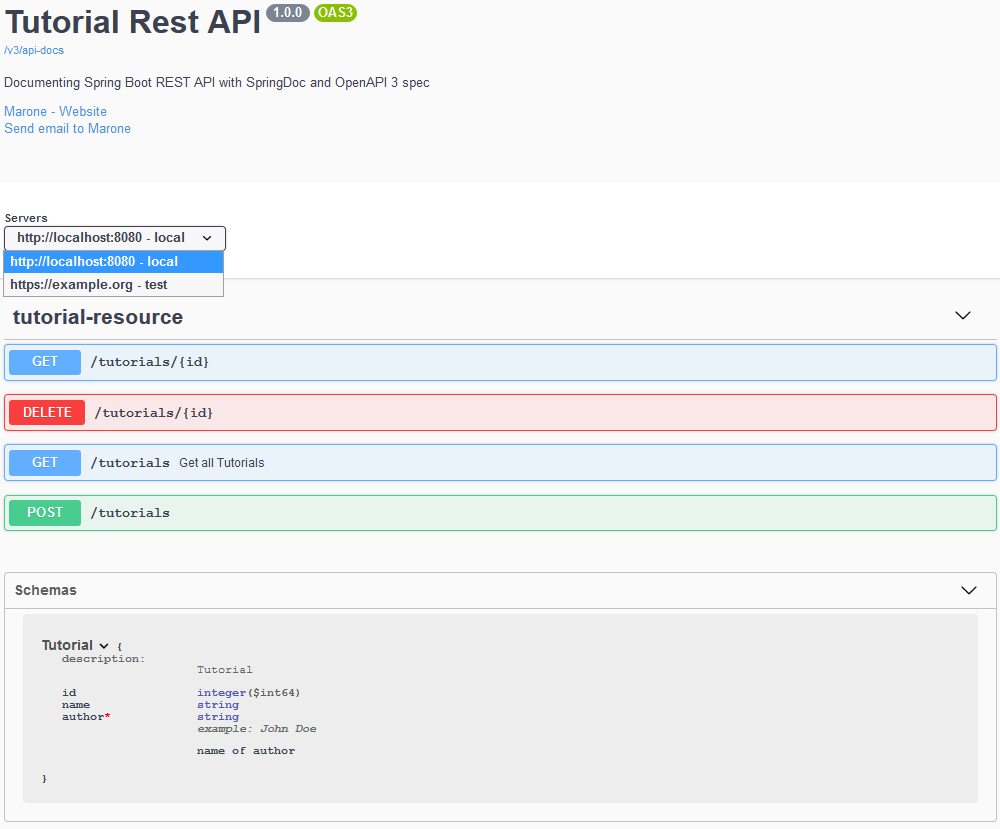